利用目的
手描きの画像からhtmlやJavaScriptのコードを作ってもらえると聞いたので試してみました。
プロンプト
(画像を添付)
画像のようなゲームで遊びたい。JavaScriptでプログラムを作って。
プロンプトのポイント
ブロック崩しの絵を描いてChatGPTに与えてみました。
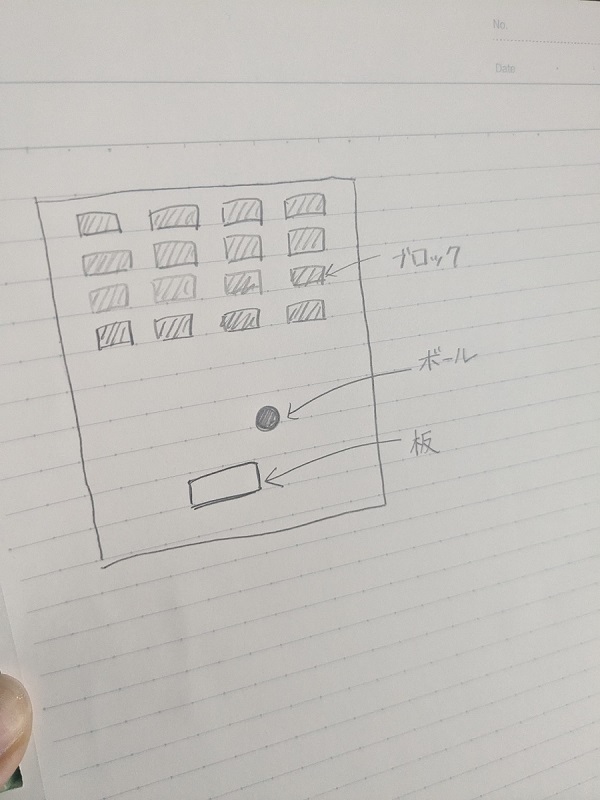
達成された成果
何の問題も無く遊べるブロック崩しゲームが出来ました。
文字ではゲームに関する情報を与えていませんが、画像から「これはブロック崩しだ」と判断したようです。
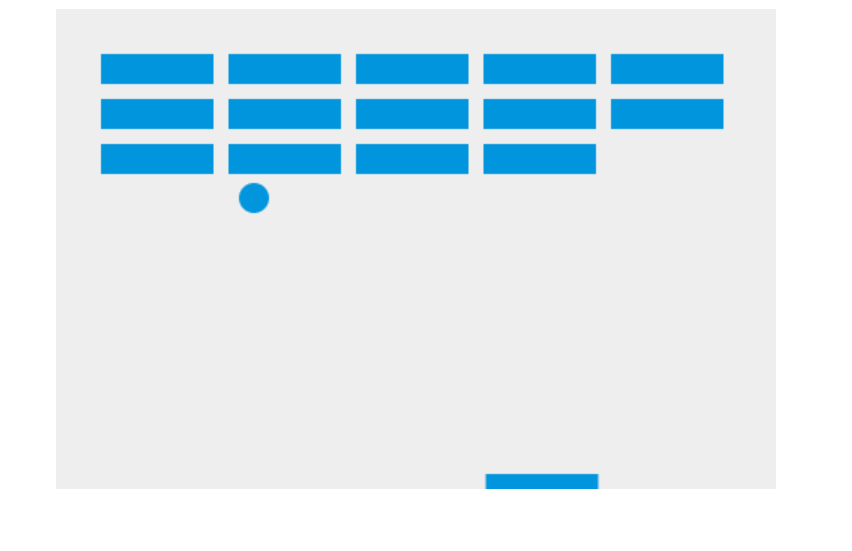
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ブロック崩しゲーム</title>
<style>
canvas {
background: #eee;
display: block;
margin: 0 auto;
}
#gameOverMessage {
display: none;
text-align: center;
font-size: 24px;
color: red;
}
</style>
</head>
<body>
<canvas id="myCanvas" width="480" height="320"></canvas>
<div id="gameOverMessage">GAME OVER</div>
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
const gameOverMessage = document.getElementById("gameOverMessage");
let ballRadius = 10;
let x = canvas.width / 2;
let y = canvas.height - 30;
let dx = 2;
let dy = -2;
const paddleHeight = 10;
const paddleWidth = 75;
let paddleX = (canvas.width - paddleWidth) / 2;
let rightPressed = false;
let leftPressed = false;
const brickRowCount = 3;
const brickColumnCount = 5;
const brickWidth = 75;
const brickHeight = 20;
const brickPadding = 10;
const brickOffsetTop = 30;
const brickOffsetLeft = 30;
const bricks = [];
for (let c = 0; c < brickColumnCount; c++) {
bricks[c] = [];
for (let r = 0; r < brickRowCount; r++) {
bricks[c][r] = { x: 0, y: 0, status: 1 };
}
}
let score = 0;
document.addEventListener("keydown", keyDownHandler, false);
document.addEventListener("keyup", keyUpHandler, false);
function keyDownHandler(e) {
if (e.key == "Right" || e.key == "ArrowRight") {
rightPressed = true;
} else if (e.key == "Left" || e.key == "ArrowLeft") {
leftPressed = true;
}
}
function keyUpHandler(e) {
if (e.key == "Right" || e.key == "ArrowRight") {
rightPressed = false;
} else if (e.key == "Left" || e.key == "ArrowLeft") {
leftPressed = false;
}
}
function collisionDetection() {
for (let c = 0; c < brickColumnCount; c++) {
for (let r = 0; r < brickRowCount; r++) {
const b = bricks[c][r];
if (b.status == 1) {
if (x > b.x && x < b.x + brickWidth && y > b.y && y < b.y + brickHeight) {
dy = -dy;
b.status = 0;
score++;
if (score == brickRowCount * brickColumnCount) {
showWinMessage();
}
}
}
}
}
}
function showWinMessage() {
gameOverMessage.textContent = "You win, congratulations!";
gameOverMessage.style.display = "block";
setTimeout(() => {
document.location.reload();
}, 2000);
}
function showGameOverMessage() {
gameOverMessage.style.display = "block";
setTimeout(() => {
document.location.reload();
}, 2000);
}
function drawBall() {
ctx.beginPath();
ctx.arc(x, y, ballRadius, 0, Math.PI * 2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
function drawPaddle() {
ctx.beginPath();
ctx.rect(paddleX, canvas.height - paddleHeight, paddleWidth, paddleHeight);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
function drawBricks() {
for (let c = 0; c < brickColumnCount; c++) {
for (let r = 0; r < brickRowCount; r++) {
if (bricks[c][r].status == 1) {
const brickX = (c * (brickWidth + brickPadding)) + brickOffsetLeft;
const brickY = (r * (brickHeight + brickPadding)) + brickOffsetTop;
bricks[c][r].x = brickX;
bricks[c][r].y = brickY;
ctx.beginPath();
ctx.rect(brickX, brickY, brickWidth, brickHeight);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
}
}
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBricks();
drawBall();
drawPaddle();
collisionDetection();
if (x + dx > canvas.width - ballRadius || x + dx < ballRadius) {
dx = -dx;
}
if (y + dy < ballRadius) {
dy = -dy;
} else if (y + dy > canvas.height - ballRadius) {
if (x > paddleX && x < paddleX + paddleWidth) {
dy = -dy;
} else {
showGameOverMessage();
return;
}
}
if (rightPressed && paddleX < canvas.width - paddleWidth) {
paddleX += 7;
} else if (leftPressed && paddleX > 0) {
paddleX -= 7;
}
x += dx;
y += dy;
requestAnimationFrame(draw);
}
draw();
</script>
</body>
</html>
使用上のヒントやアドバイス
「ボールの移動速度を6倍にしてほしい」等のカスタムにも答えてくれます。
レビューを書く | |
AIアセットラボ
Average rating: 0 reviews